順次ステップによるワークフロー
ワークフローは、特定の順序で次々と連鎖して実行することができます。
制御フローダイアグラム
この例では、then
メソッドを使ってワークフローのステップを連結し、順番に実行しながらデータを受け渡す方法を示しています。
制御フローダイアグラムは以下の通りです:
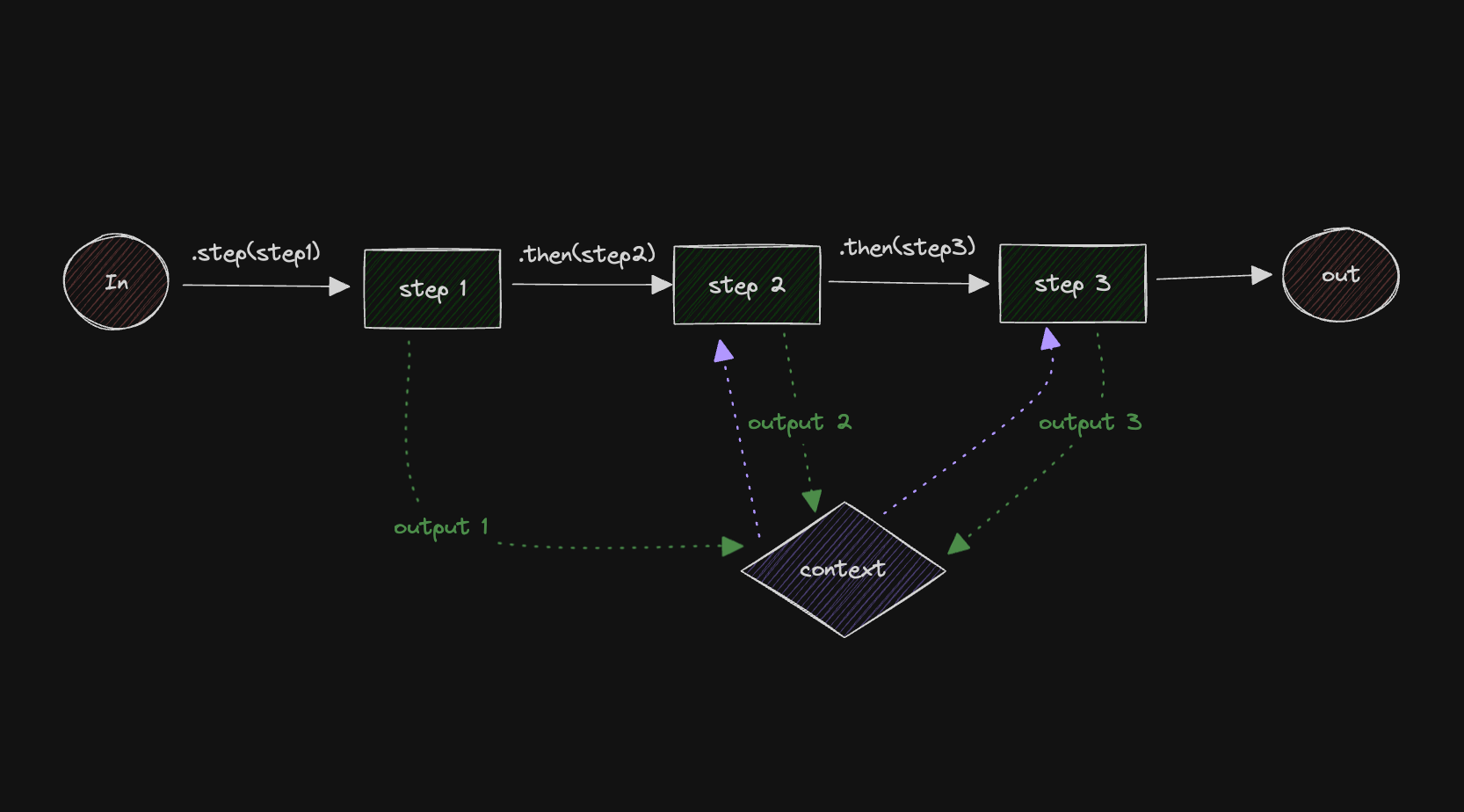
ステップの作成
まず、ステップを作成し、ワークフローを初期化しましょう。
import { Step, Workflow } from "@mastra/core/workflows";
import { z } from "zod";
const stepOne = new Step({
id: "stepOne",
execute: async ({ context }) => ({
doubledValue: context.triggerData.inputValue * 2,
}),
});
const stepTwo = new Step({
id: "stepTwo",
execute: async ({ context }) => {
if (context.steps.stepOne.status !== "success") {
return { incrementedValue: 0 };
}
return { incrementedValue: context.steps.stepOne.output.doubledValue + 1 };
},
});
const stepThree = new Step({
id: "stepThree",
execute: async ({ context }) => {
if (context.steps.stepTwo.status !== "success") {
return { tripledValue: 0 };
}
return { tripledValue: context.steps.stepTwo.output.incrementedValue * 3 };
},
});
// Build the workflow
const myWorkflow = new Workflow({
name: "my-workflow",
triggerSchema: z.object({
inputValue: z.number(),
}),
});
ステップを連結してワークフローを実行する
それでは、ステップを順番につなげてみましょう。
// sequential steps
myWorkflow.step(stepOne).then(stepTwo).then(stepThree);
myWorkflow.commit();
const { start } = myWorkflow.createRun();
const res = await start({ triggerData: { inputValue: 90 } });
GitHubで例を見る