分岐パス
データを処理する際、中間結果に基づいて異なる処理を行う必要がよくあります。この例では、ワークフローが複数のパスに分岐し、それぞれのパスが前のステップの出力に基づいて異なる処理を実行する方法を示します。
フロー制御ダイアグラム
この例では、ワークフローが分岐し、それぞれのパスが前のステップの出力に基づいて異なる処理を実行する方法を示しています。
フロー制御ダイアグラムは次のとおりです:
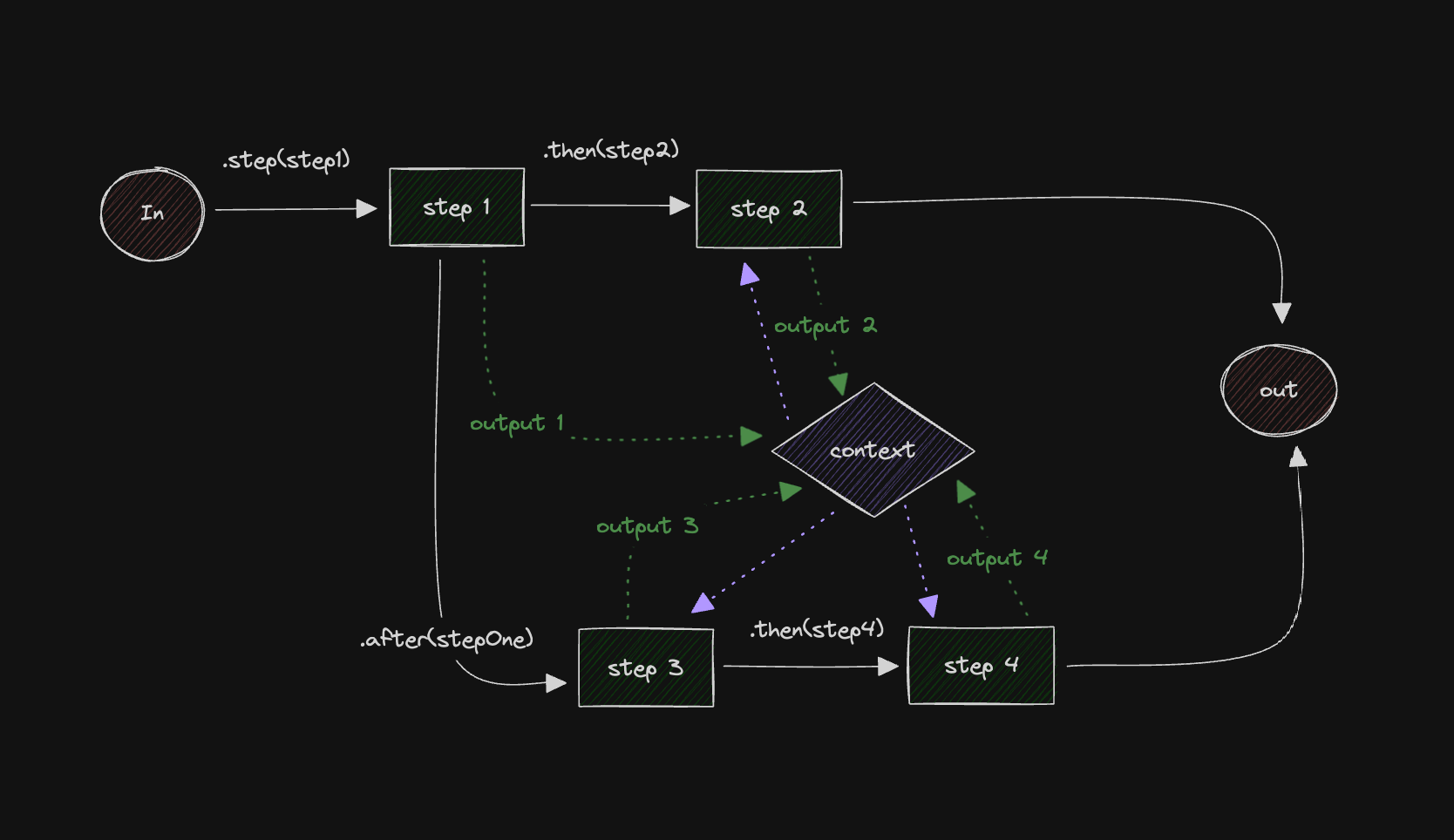
ステップの作成
まず、ステップを作成し、ワークフローを初期化しましょう。
import { Step, Workflow } from "@mastra/core/workflows";
import { z } from "zod"
const stepOne = new Step({
id: "stepOne",
execute: async ({ context }) => ({
doubledValue: context.triggerData.inputValue * 2
})
});
const stepTwo = new Step({
id: "stepTwo",
execute: async ({ context }) => {
const stepOneResult = context.getStepResult<{ doubledValue: number }>("stepOne");
if (!stepOneResult) {
return { isDivisibleByFive: false }
}
return { isDivisibleByFive: stepOneResult.doubledValue % 5 === 0 }
}
});
const stepThree = new Step({
id: "stepThree",
execute: async ({ context }) =>{
const stepOneResult = context.getStepResult<{ doubledValue: number }>("stepOne");
if (!stepOneResult) {
return { incrementedValue: 0 }
}
return { incrementedValue: stepOneResult.doubledValue + 1 }
}
});
const stepFour = new Step({
id: "stepFour",
execute: async ({ context }) => {
const stepThreeResult = context.getStepResult<{ incrementedValue: number }>("stepThree");
if (!stepThreeResult) {
return { isDivisibleByThree: false }
}
return { isDivisibleByThree: stepThreeResult.incrementedValue % 3 === 0 }
}
});
// New step that depends on both branches
const finalStep = new Step({
id: "finalStep",
execute: async ({ context }) => {
// Get results from both branches using getStepResult
const stepTwoResult = context.getStepResult<{ isDivisibleByFive: boolean }>("stepTwo");
const stepFourResult = context.getStepResult<{ isDivisibleByThree: boolean }>("stepFour");
const isDivisibleByFive = stepTwoResult?.isDivisibleByFive || false;
const isDivisibleByThree = stepFourResult?.isDivisibleByThree || false;
return {
summary: `The number ${context.triggerData.inputValue} when doubled ${isDivisibleByFive ? 'is' : 'is not'} divisible by 5, and when doubled and incremented ${isDivisibleByThree ? 'is' : 'is not'} divisible by 3.`,
isDivisibleByFive,
isDivisibleByThree
}
}
});
// Build the workflow
const myWorkflow = new Workflow({
name: "my-workflow",
triggerSchema: z.object({
inputValue: z.number(),
}),
});
分岐パスとステップの連結
それでは、分岐パスを持つワークフローを設定し、複合的な .after([])
構文を使ってそれらを統合してみましょう。
// Create two parallel branches
myWorkflow
// First branch
.step(stepOne)
.then(stepTwo)
// Second branch
.after(stepOne)
.step(stepThree)
.then(stepFour)
// Merge both branches using compound after syntax
.after([stepTwo, stepFour])
.step(finalStep)
.commit();
const { start } = myWorkflow.createRun();
const result = await start({ triggerData: { inputValue: 3 } });
console.log(result.steps.finalStep.output.summary);
// Output: "The number 3 when doubled is not divisible by 5, and when doubled and incremented is divisible by 3."
高度なブランチとマージ
複数のブランチやマージポイントを使って、より複雑なワークフローを作成できます。
const complexWorkflow = new Workflow({
name: "complex-workflow",
triggerSchema: z.object({
inputValue: z.number(),
}),
});
// Create multiple branches with different merge points
complexWorkflow
// Main step
.step(stepOne)
// First branch
.then(stepTwo)
// Second branch
.after(stepOne)
.step(stepThree)
.then(stepFour)
// Third branch (another path from stepOne)
.after(stepOne)
.step(
new Step({
id: "alternativePath",
execute: async ({ context }) => {
const stepOneResult = context.getStepResult<{ doubledValue: number }>(
"stepOne",
);
return {
result: (stepOneResult?.doubledValue || 0) * 3,
};
},
}),
)
// Merge first and second branches
.after([stepTwo, stepFour])
.step(
new Step({
id: "partialMerge",
execute: async ({ context }) => {
const stepTwoResult = context.getStepResult<{
isDivisibleByFive: boolean;
}>("stepTwo");
const stepFourResult = context.getStepResult<{
isDivisibleByThree: boolean;
}>("stepFour");
return {
intermediateResult: "Processed first two branches",
branchResults: {
branch1: stepTwoResult?.isDivisibleByFive,
branch2: stepFourResult?.isDivisibleByThree,
},
};
},
}),
)
// Final merge of all branches
.after(["partialMerge", "alternativePath"])
.step(
new Step({
id: "finalMerge",
execute: async ({ context }) => {
const partialMergeResult = context.getStepResult<{
intermediateResult: string;
branchResults: { branch1: boolean; branch2: boolean };
}>("partialMerge");
const alternativePathResult = context.getStepResult<{ result: number }>(
"alternativePath",
);
return {
finalResult: "All branches processed",
combinedData: {
fromPartialMerge: partialMergeResult?.branchResults,
fromAlternativePath: alternativePathResult?.result,
},
};
},
}),
)
.commit();
GitHubで例を見る