Using with CopilotKit in React
CopilotKit provides React components to quickly integrate customizable AI copilots into your application. Combined with Mastra, you can build sophisticated AI apps featuring bidirectional state synchronization and interactive UIs.
Create a Mastra Project
Create a new Mastra project using the mastra
CLI:
npx
npx create-mastra@latest
Select the agent example when scaffolding your project. This will give you a weather agent.
For detailed setup instructions, see the installation guide.
Basic Setup
Integrating Mastra with CopilotKit involves two main steps: setting up the backend runtime and configuring your frontend components.
npm
npm install @copilotkit/runtime
Set up the runtime
You can leverage Mastra’s custom API routes to add CopilotKit’s runtime to your Mastra server.
The current version of the integration leverages MastraClient
to format Mastra agents into the AGUI format of CopilotKit.
npm
npm install @mastra/client-js
Next, let’s update the Mastra instance with a custom API route for CopilotKit.
import { Mastra } from '@mastra/core/mastra';
import { createLogger } from '@mastra/core/logger';
import { LibSQLStore } from '@mastra/libsql';
import { registerApiRoute } from '@mastra/core/server';
import {
CopilotRuntime,
copilotRuntimeNodeHttpEndpoint,
ExperimentalEmptyAdapter
} from '@copilotkit/runtime';
import { MastraClient } from '@mastra/client-js';
import { weatherAgent } from './agents';
const serviceAdapter = new ExperimentalEmptyAdapter();
export const mastra = new Mastra({
agents: { weatherAgent },
storage: new LibSQLStore({
// stores telemetry, evals, ... into memory storage,
// if you need to persist, change to file:../mastra.db
url: ":memory:",
}),
logger: createLogger({
name: 'Mastra',
level: 'info',
}),
server: {
apiRoutes: [
registerApiRoute('/copilotkit', {
method: `POST`,
handler: async (c) => {
// N.B. Current integration leverages MastraClient to fetch AGUI.
// Future versions will support fetching AGUI from mastra context.
const client = new MastraClient({
baseUrl: 'http://localhost:4111'
});
const runtime = new CopilotRuntime({
agents: await client.getAGUI({ resourceId: 'weatherAgent' })
});
const handler = copilotRuntimeNodeHttpEndpoint({
endpoint: "/copilotkit",
runtime,
serviceAdapter,
});
return handler.handle(c.req.raw, {})
}
})
]
}
});
With this setup you now have CopilotKit running on your Mastra server. You can start your Mastra server with mastra dev
.
Set up the UI
Install CopilotKit’s React components:
npm
npm install @copilotkit/react-core @copilotkit/react-ui
Next, add CopilotKit’s React components to your frontend.
import { CopilotChat } from "@copilotkit/react-ui";
import { CopilotKit } from "@copilotkit/react-core";
import "@copilotkit/react-ui/styles.css";
export function CopilotKitComponent() {
return (
<CopilotKit
runtimeUrl="http://localhost:4111/copilotkit"
agent="weatherAgent"
>
<CopilotChat
labels={{
title: "Your Assistant",
initial: "Hi! 👋 How can I assist you today?",
}}
/>
</CopilotKit>
)
}
Render the component and start building the future!
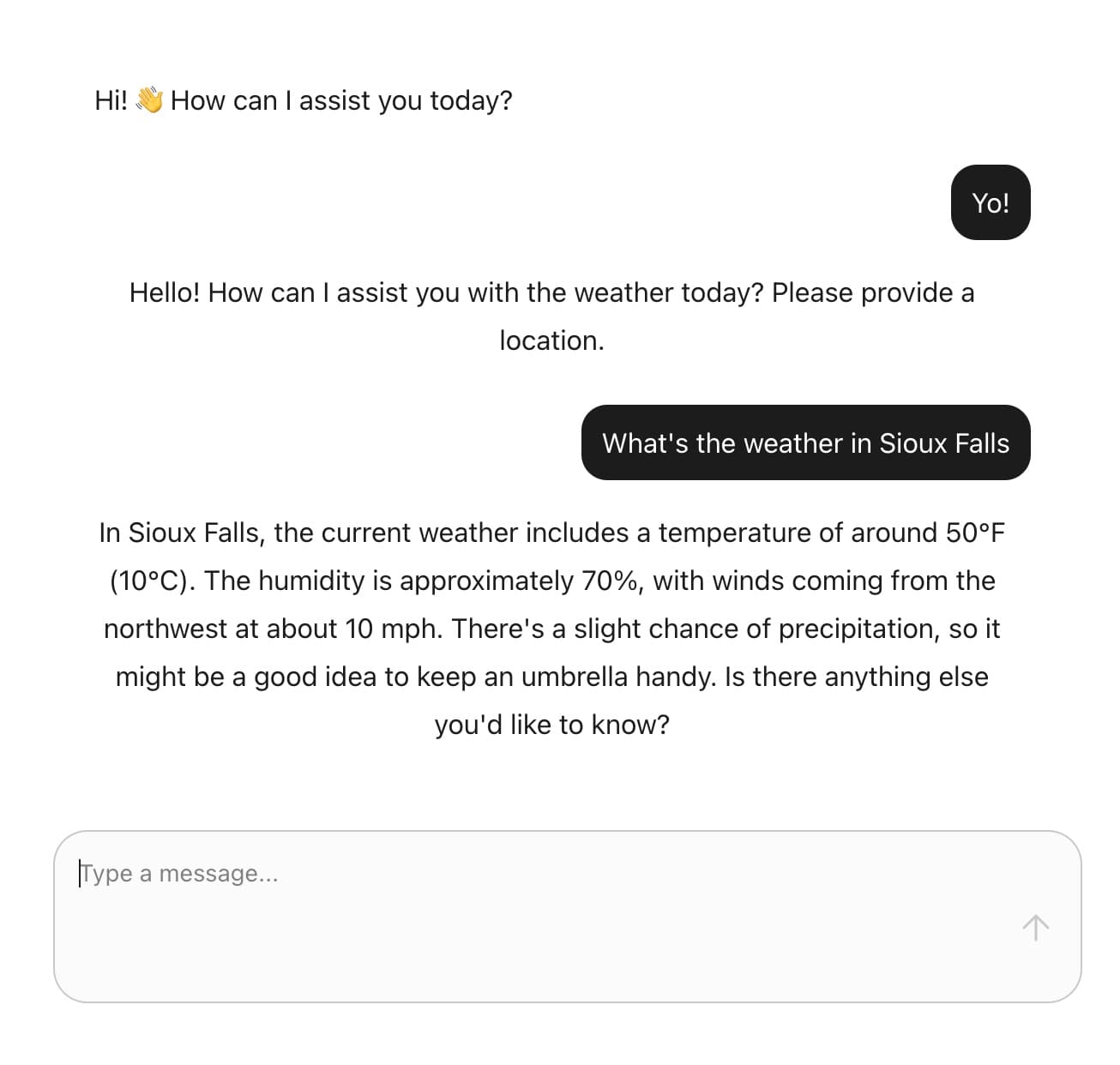