At Mastra, we love agents. What we’ve found is that agents are even more powerful when you pair them with workflows when you need more structured decision-making.
We’re hardly the first to discover this — Anthropic had a great blog post on this last month.
Enter workflows: a way to explicitly structure and determine how agents and LLMs work together.
Workflows let you not only control the general flow of task-execution but also add checkpoints, moments when computation is suspended (so a human can provide feedback or guidance to the agent) before the workflow is resumed and ultimately completed.
Mastra workflow patterns
We’ve built several workflow patterns in Mastra that you can add to projects and customize.
Think of these as tools for the implementing most common types of workflows:
Sequential chains
Sequential chains are useful for organizing steps that need to be executed one after another in a specific order. But they’re more than just ordered execution.
These chains can ensure that each step's output becomes the input for the next step—which is particularly helpful when you want to control data flows and/or manage dependencies.
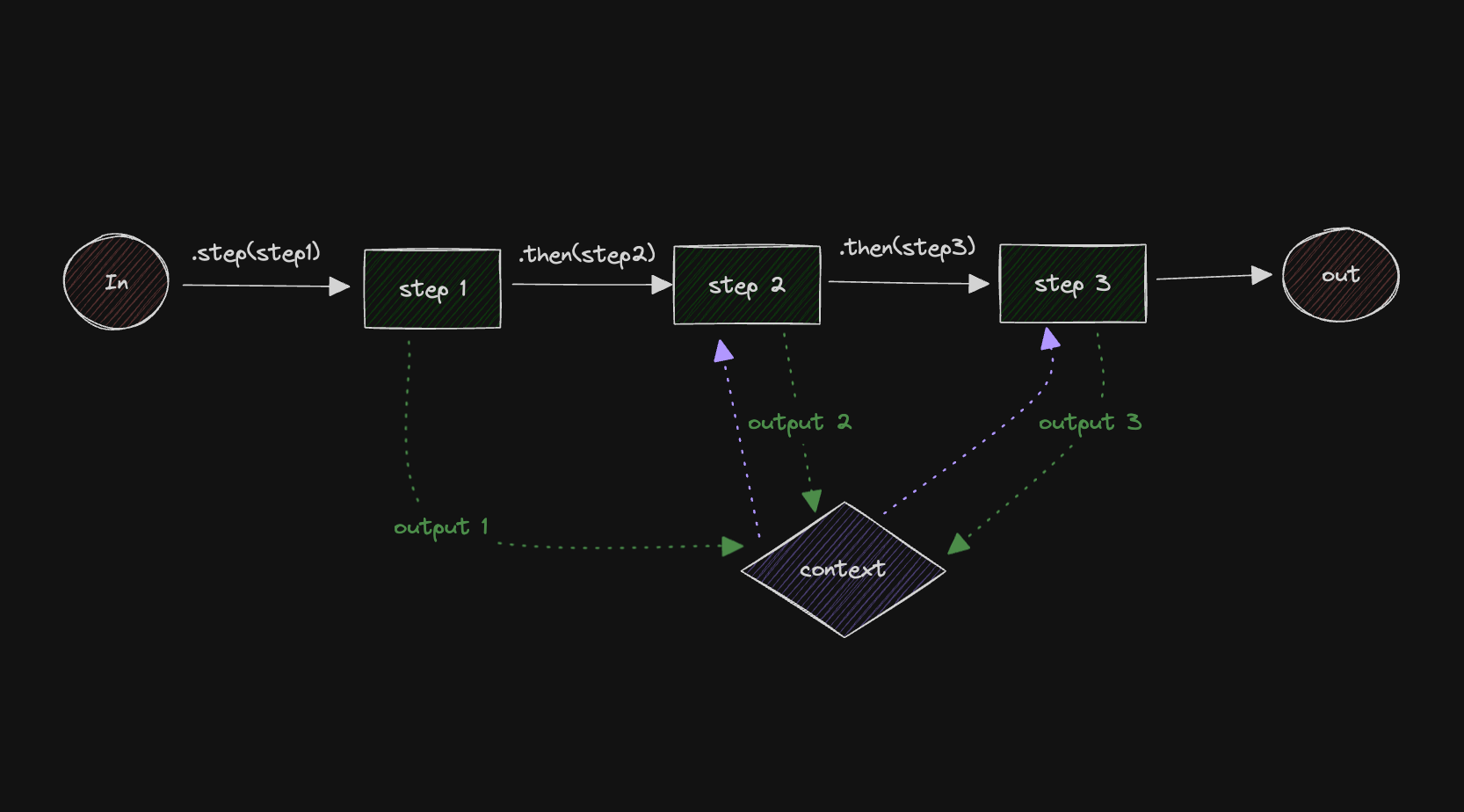
Sequential chains, where steps follow a linear order, are the “hello world” of workflows.
They're valuable for:
- Simple document processing pipelines: Extracting, validating, and storing text or data in a structured sequence.
- Multi-stage approval processes: Ensuring documents or requests pass through each level of review in order.
- Data transformation and validation sequences: Cleaning, transforming, and verifying data step by step.
We've built a simple code example for building sequential chains in Mastra.
The relevant bit:
myWorkflow.step(stepOne).then(stepTwo).then(stepThree);
In the example, stepTwo
only executes after stepOne
succeeds, and stepThree
only executes after stepTwo
succeeds.
If you want to dive deeper, here are the docs.
Parallel chains
Parallel chains are ideal for tasks that can be executed simultaneously (rather than one after another.) These workflows enable multiple steps to run independently, reducing overall processing time. Unlike sequential chains, parallel chains don't rely on the output of one step to feed into the next. Instead, tasks run concurrently and can converge later if needed.
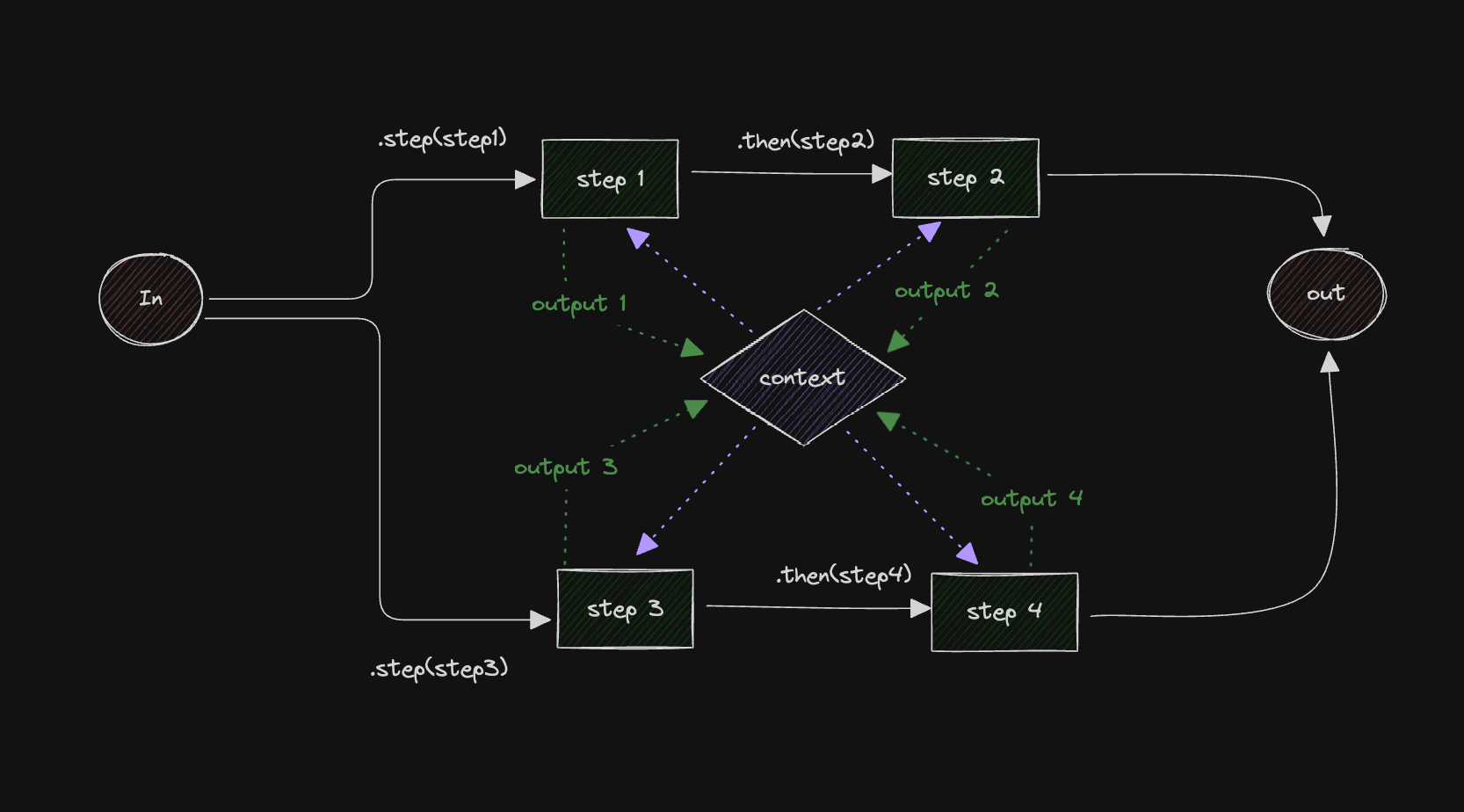
From the user’s perspective, parallel execution reduces latency; from a system perspective, it improves resource utilization. Key applications include:
- Batch processing: Handling multiple documents, datasets, or files at once.
- Concurrent data analysis: Running independent computations on the same or different datasets simultaneously.
- Multitask workflows: Managing distinct but related tasks, like generating reports while sending notifications.
We've built a simple code example for building parallel chains in Mastra.
The relevant bit:
myWorkflow.step(stepOne).then(stepTwo).step(stepThree).then(stepFour);
This creates a workflow where stepOne
and stepThree
kick off their sequential chains concurrently. Each chain also updates the global context with its results. The overall workflow engine manages dependencies and ensures that all parallel steps are completed.
You can read more about parallel workflows here.
Subscribed chains
Subscribed chains are much like parallel chains—except they’re only triggered by the completion of a specific step. In other words, they’re event-driven.
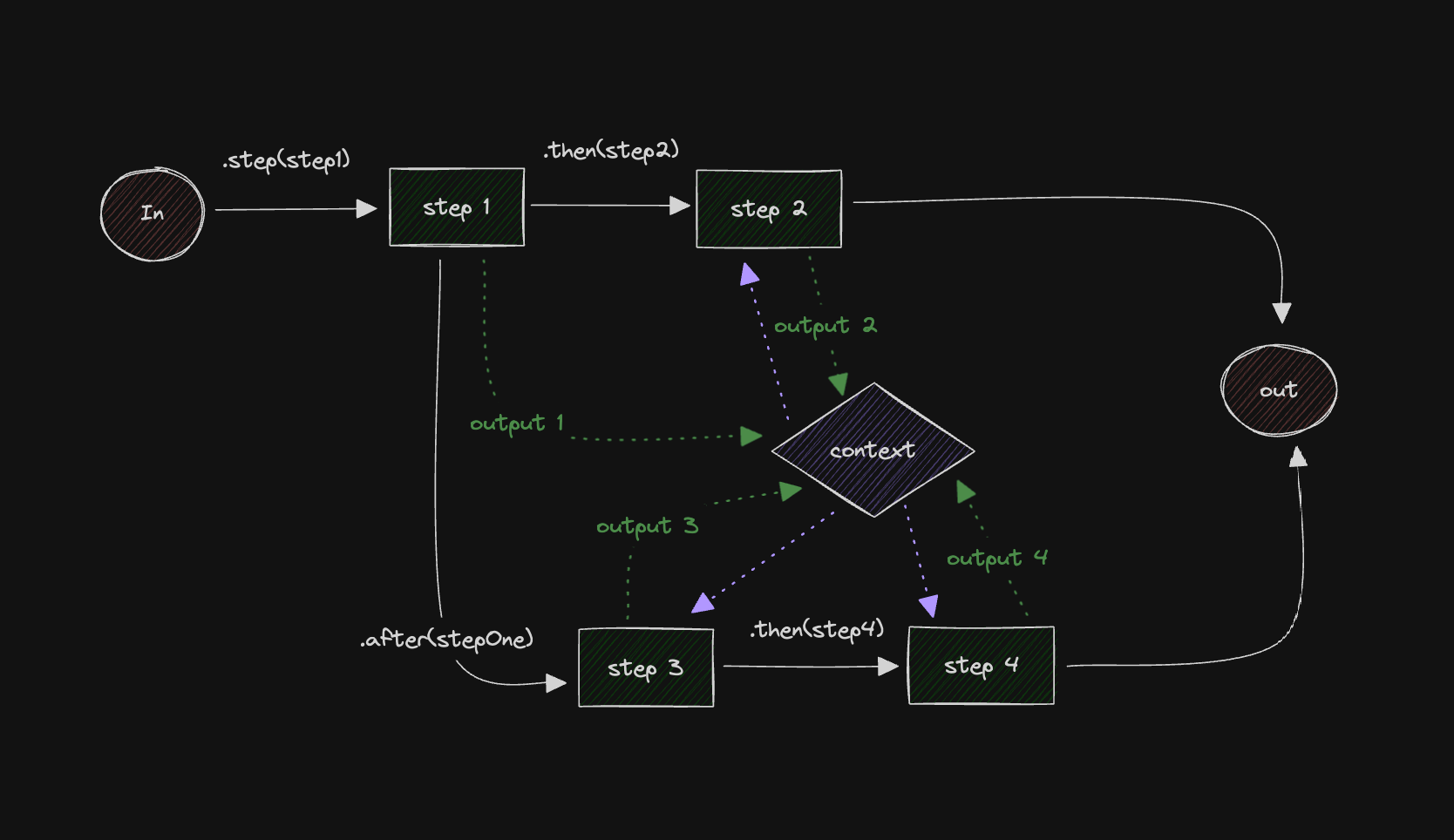
Subscribed chains are powerful for managing workflows that require simultaneous but event-driven actions like:
- Complex notifications: Sending different alerts to multiple channels using specific triggers.
- Audit trail generation: Logging actions and changes in parallel to the main workflow.
- Asynchronous processing: Handling background tasks like data enrichment without blocking the main process.
- Secondary validation: Running additional checks alongside the primary workflow.
We've built a simple code example for building subscribed chains in Mastra.
The relevant bit:
myWorkflow
.step(stepOne)
.then(stepTwo)
.after(stepOne)
.step(stepThree)
.then(stepFour)
.commit();
Read more about subscriber chains here
Suspending and resuming workflows
For workflows that require human-in-the-loop, have external event dependencies, or involve operations that are too long to be kept in memory, Mastra has tools for pausing execution, persisting state, and resuming execution.
Here are some examples of human-in-the-loop workflows that benefit from pausing and resuming execution:
- Approval workflows: A workflow generates a document that require approval before moving to the next step. The process suspends until the manager approves or rejects the document.
- Customer service escalation: An AI chatbot handles initial customer inquiries but escalates complex issues to a human agent. The human specifies a solution and delegates back to the AI agent to complete follow-up actions.
Use suspend()
to ‘pause’ any Mastra workflow. When called, suspend()
triggers a cascade of state-management operations:
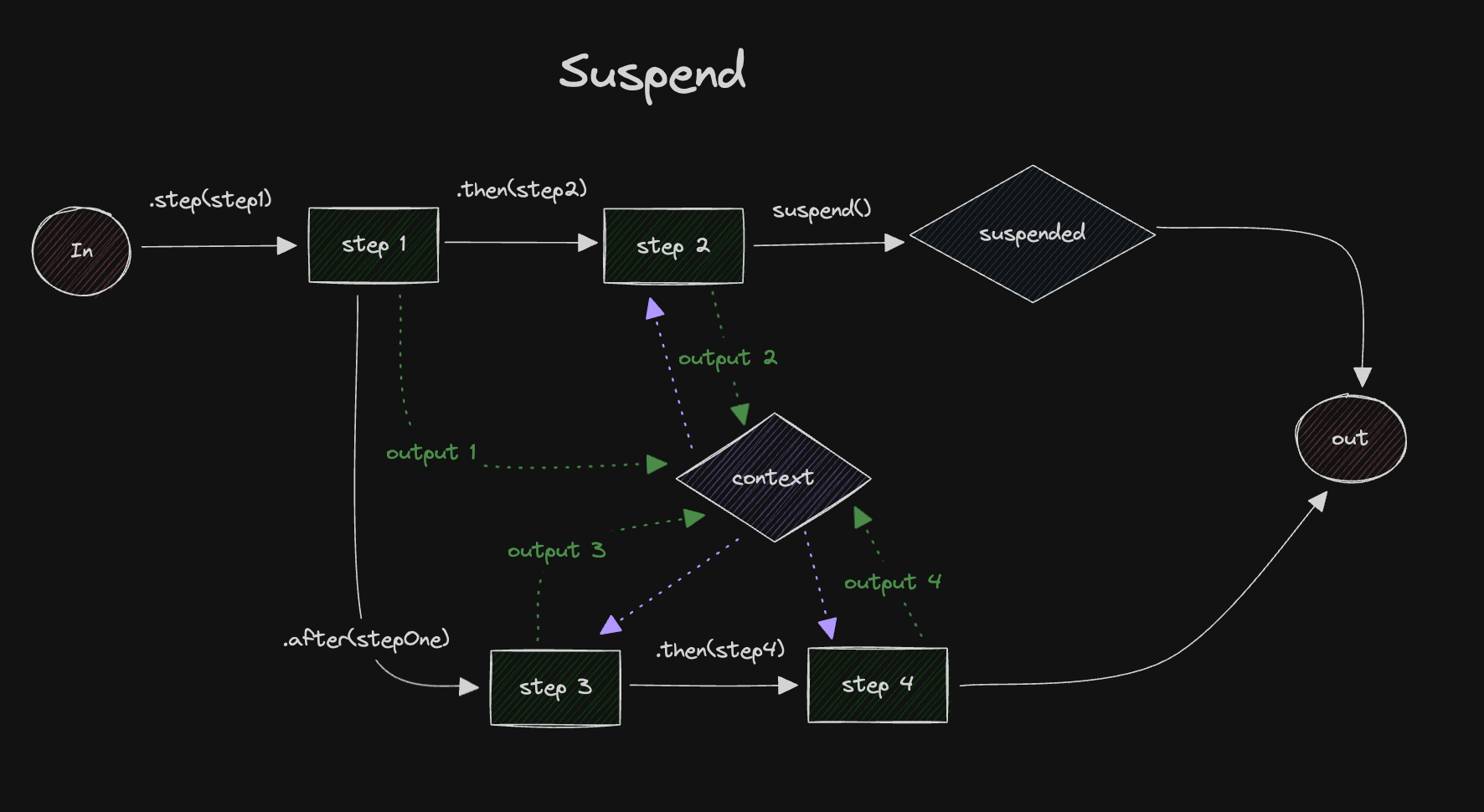
Here’s an example of how to use suspend()
in Mastra:
execute: async ({ suspend, context }) => {
const needsApproval = await checkApprovalRequired();
if (needsApproval) {
await suspend(); // Workflow pauses here
return { status: "awaiting_approval" };
}
};
Once a Mastra workflow is suspended, it can be resumed using resume()
. When called, resume()
will continue executing the workflow from where it last left off.
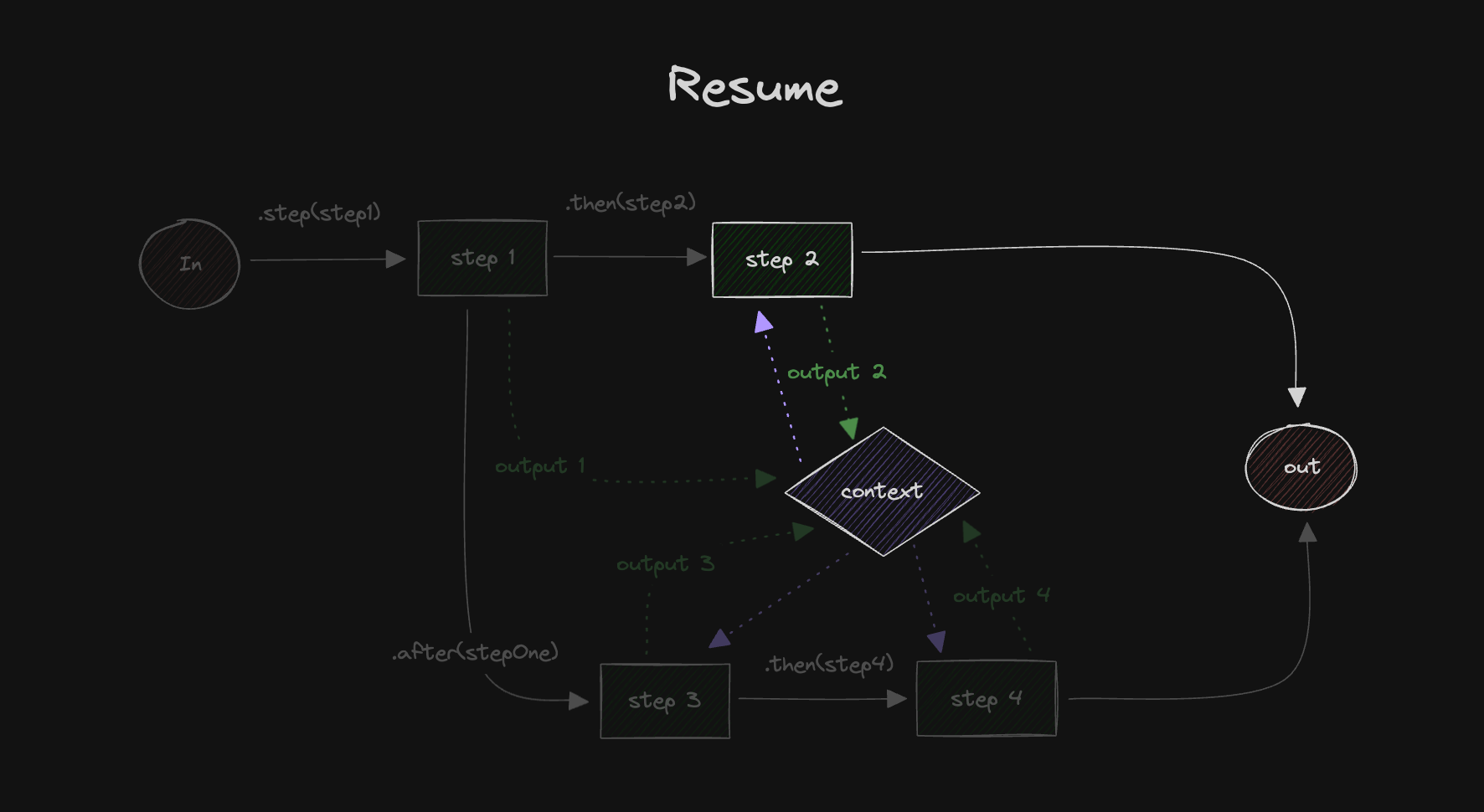
The resume()
function can be called from within your application after you handle any stipulated human-in-the-loop interactions. You can pass that external context back into the workflow, trusting that it will pick up where it left off.
Here’s an example of using resume()
with Mastra:
const workflow = mastra.getWorkflow("myWorkflow");
const { runId, start } = workflow.createRun();
await start();
await workflow.watch(runId, {
onTransition: async ({ activePaths, context }) => {
for (const path of activePaths) {
const ctx = context.stepResults?.[path.stepId]?.status;
if (ctx === "suspended") {
// Handle suspension logic here.
if (confirmed) {
await workflow.resume({
stepId: path.stepId,
runId,
context: {
confirm: true,
},
});
}
}
}
},
});
What’s next: orchestrator-workers
In the near future, we plan to introduce hierarchal workflow management through orchestrator-workers. Here’s a high-level sneak peek at what that looks like:
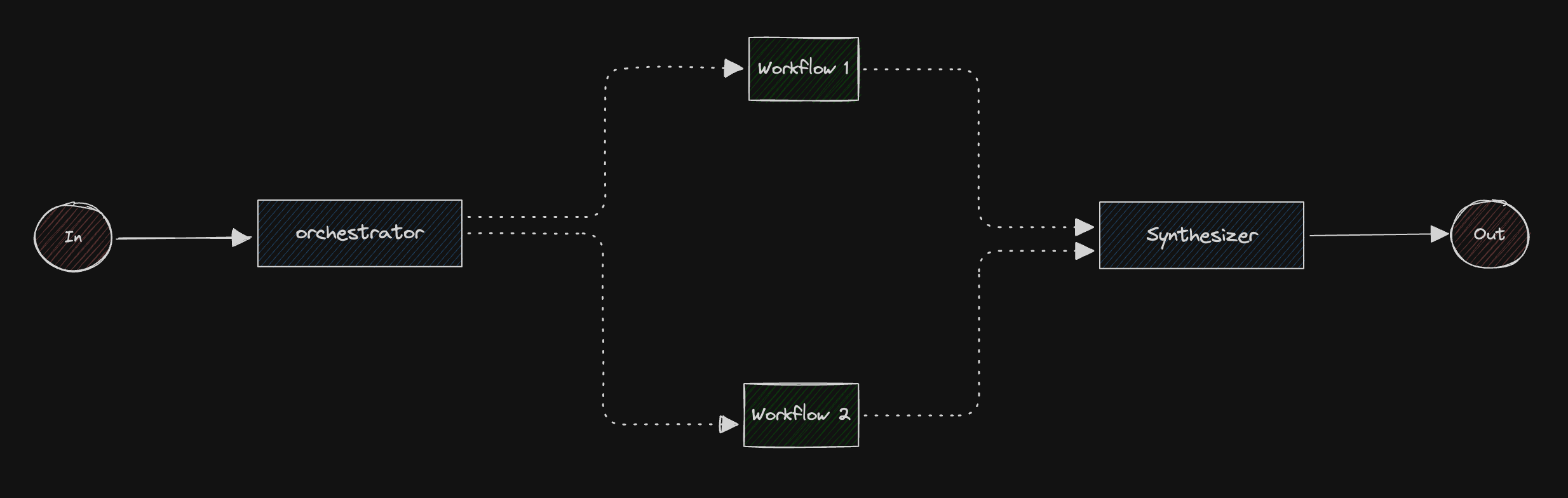
Orchestrator-workers will let you compose and manage multiple workflows.
Stay tuned!