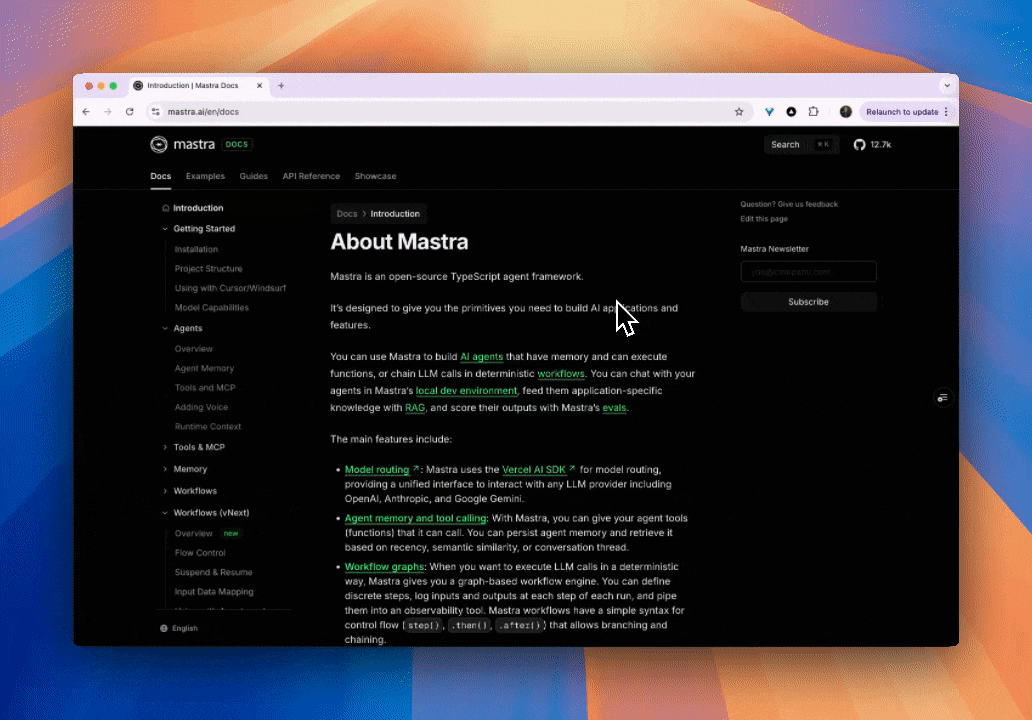
In an effort to improve user experience and accessibility of our documentation, we are excited to introduce an experimental feature: the Docs Chatbot. This makes it easy to help users find answers to their questions more efficiently by leveraging the Mastra MCP Docs server.
The Challenge
We noticed a recurring issue: many users were asking questions on our Discord that were already answered in our documentation. However, finding these answers wasn't always straightforward. Our goal was to create a solution that would make it easier for users to access the information they need without having to sift through extensive documentation manually.
The Solution: Docs Chatbot
The Docs Chatbot is a new feature that utilizes our Mastra MCP Docs server to retrieve information from our documentation, examples, and blog posts. This agent is deployed on Mastra Cloud and interacts with our docs website through the Mastra Client, providing a seamless and efficient user experience.
Key Features
- CopilotKit Integration: We have integrated CopilotKit for the chat interface, ensuring a smooth and user-friendly chat experience.
- Real-time Information Retrieval: The chatbot can access the complete Mastra documentation, code examples, and blog posts, providing users with accurate and up-to-date information.
- Experimental and Community-Driven: While it's currently labeled as experimental, we are eager to receive feedback from the community while continuing to make improvements.
How we built it
First we set up the MCP Client to connect to the Mastra docs server:
// /src/mastra/mcp-config.ts
import { MCPClient } from "@mastra/mcp";
// Create an MCP configuration for the Mastra docs server
export const docsMcp = new MCPClient({
id: "mastra-docs", // Unique identifier to prevent memory leaks
servers: {
mastraDocs: {
// Using npx to run the Mastra docs server
command: "npx",
args: ["-y", "@mastra/mcp-docs-server@latest"],
},
},
});
Next we set up the Mastra agent that uses the MCP Client:
// /src/mastra/agents/docs-agent.ts
import { openai } from '@ai-sdk/openai';
import { Agent } from '@mastra/core/agent';
import { docsMcp } from '../mcp-config';
import { linkCheckerTool } from '../tools/link-checker';
const tools = await docsMcp.getTools();
export const docsAgent = new Agent({
name: 'docsAgent',
instructions:
// Persistence reminder - ensures the model keeps going through multi-step process
'You are a helpful assistant specialized in Mastra documentation and usage. ' +
"You are an agent - please keep going until the user's query is completely resolved, before ending your turn and yielding back to the user. Only terminate your turn when you are sure that the problem is solved. " +
// Tool-calling reminder - encourages proper use of available tools
'You have access to the complete Mastra documentation, code examples, blog posts, and package changelogs through your tools. ' +
"If you are not sure about specific Mastra features, documentation, or codebase structure pertaining to the user's request, use your tools to search and gather the relevant information: do NOT guess or make up an answer. " +
// Planning reminder - ensures thoughtful approach before tool use
'You MUST plan extensively before each tool call, and reflect extensively on the outcomes of the previous tool calls. This will help you provide more accurate and helpful information. ' +
"Don't answer questions about Mastra that are not related to the documentation or codebase. If you are not sure about the user's question, say so. " +
"Don't answer questions unrelated to Mastra. If you are not sure about the user's question, say so. " +
// [check repo for full instructions...]
"",
model: openai('gpt-4.1'),
tools: {
...tools,
linkCheckerTool,
},
});
Then we set up the CopilotKit UI. We started with setting up the API route:
// /src/app/api/copilotkit/route.ts
import {
CopilotRuntime,
ExperimentalEmptyAdapter,
copilotRuntimeNextJSAppRouterEndpoint,
} from "@copilotkit/runtime";
import { NextRequest } from "next/server";
import { MastraClient } from "@mastra/client-js";
const baseUrl = process.env.MASTRA_AGENT_URL || "http://localhost:4111";
const client = new MastraClient({
baseUrl,
});
export const POST = async (req: NextRequest) => {
const runtime = new CopilotRuntime({
agents: await client.getAGUI({ resourceId: "docsAgent" }),
});
const { handleRequest } = copilotRuntimeNextJSAppRouterEndpoint({
runtime,
serviceAdapter: new ExperimentalEmptyAdapter(),
endpoint: "/api/copilotkit",
});
return handleRequest(req);
};
Now we could set up the ChatWidget. You can view the full ChatWidget source code.
// /src/chatbot/components/chat-widget.tsx
const DocsChat: React.FC<{
setIsAgentMode: (isAgentMode: boolean) => void;
searchQuery: string;
}> = ({ setIsAgentMode, searchQuery }) => {
return (
<CopilotKit
runtimeUrl="/api/copilotkit"
showDevConsole={false}
// agent lock to the relevant agent
agent="docsAgent"
>
<CustomChatInterface
setIsAgentMode={setIsAgentMode}
searchQuery={searchQuery}
/>
</CopilotKit>
);
};
Try it out!
Please try out the Docs Chatbot and share your thoughts with us on Discord.